The TabBar
widget in Flutter is used to create a set of horizontal tabs. Each tab can be associated with a content widget which is displayed when the tab is selected. This is particularly useful for organizing content into sections, making it easy for users to navigate through different views within the same screen.
In this tutorial, we’ll demonstrate everything about TabBar in Flutter, we will show you how to implement it in your app and we will demonstrate some of the TabBar examples.
We’ll cover all the following topics in detail:
Table of Contents
If you are a visual or practical learner you can watch a complete video of Tabar from the official Flutter channel.
How to SetUp TabBar in Flutter?
To setup TabBar in Flutter we have a minimal code for you that you can use for your work.
DefaultTabController(
length: 3,
child: Scaffold(
backgroundColor: Colors.black,
appBar: AppBar(
backgroundColor: Colors.black,
title: const Center(
child: Text(
"TabBar Demo",
style: TextStyle(color: Colors.white),
)),
bottom: const TabBar(
tabs: [
Tab(icon: Icon(Icons.home, color: Colors.white)),
Tab(icon: Icon(Icons.add_alert, color: Colors.white)),
Tab(icon: Icon(Icons.save_alt_sharp, color: Colors.white)),
],
),
),
body: const TabBarView(
children: [
Icon(
Icons.home,
color: Colors.white,
size: 140,
),
Icon(
Icons.notifications,
color: Colors.white,
size: 140,
),
Icon(
Icons.download,
color: Colors.white,
size: 140,
),
],
),
),
);
To implement TabBar we have some prerequisites to configure in our app.
1. Setup Your Flutter Project: Ensure you have a Flutter project setup. If not, you can create one using:
flutter create tabbar_example
cd tabbar_example
2. Import Necessary Packages
Import the necessary Flutter material package in your main.dart
file:
import 'package:flutter/material.dart';
3. Wrap the Scaffold widget inside the DefaultTabController. This is the most simple use case. If you want to control the tabs programmatically, then you can use TabController.
4. Place the TabBar widget in the bottom of the AppBar.
5. Place TabBarView in the body of the Scaffold. The TabBarView is just like the PagView, which is mostly used with the TabBar. The pages are shown based on the selected tab.
import 'package:flutter/material.dart';
class HomePage extends StatefulWidget {
const HomePage({super.key});
@override
State<HomePage> createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
@override
Widget build(BuildContext context) {
return DefaultTabController(
length: 3,
child: Scaffold(
backgroundColor: Colors.black,
appBar: AppBar(
backgroundColor: Colors.black,
title: const Center(
child: Text(
"TabBar Demo",
style: TextStyle(color: Colors.white),
)),
bottom: const TabBar(
tabs: [
Tab(icon: Icon(Icons.home, color: Colors.white)),
Tab(icon: Icon(Icons.add_alert, color: Colors.white)),
Tab(icon: Icon(Icons.save_alt_sharp, color: Colors.white)),
],
),
),
body: const TabBarView(
children: [
Icon(
Icons.home,
color: Colors.white,
size: 140,
),
Icon(
Icons.notifications,
color: Colors.white,
size: 140,
),
Icon(
Icons.download,
color: Colors.white,
size: 140,
),
],
),
),
);
}
}
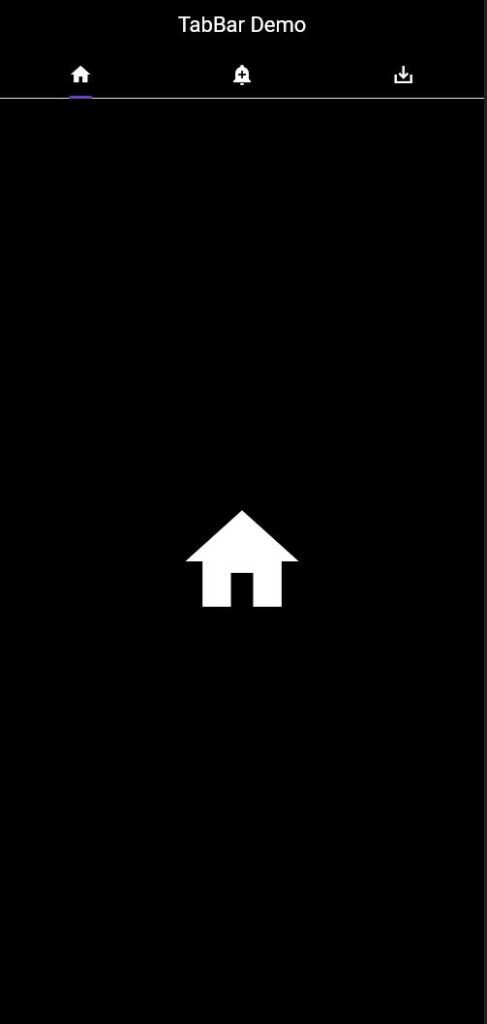
Tab Color
To change the color of a tab: we can use indicatorColor: Colors.amberAccent
TabBar(
indicatorColor: Colors.red,
tabs: []
),
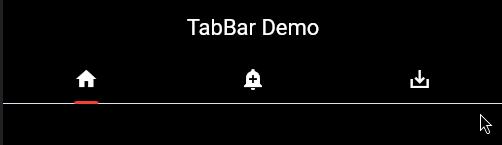
Tab Height
To change the tab height: we can use indicatorWeight: 8,
TabBar(
indicatorWeight: 8,
tabs: []
),
Change the indicator design
To achieve this we can use
TabBar(
indicator: BoxDecoration(
color: Colors.red,
borderRadius: BorderRadius.circular(20)
)
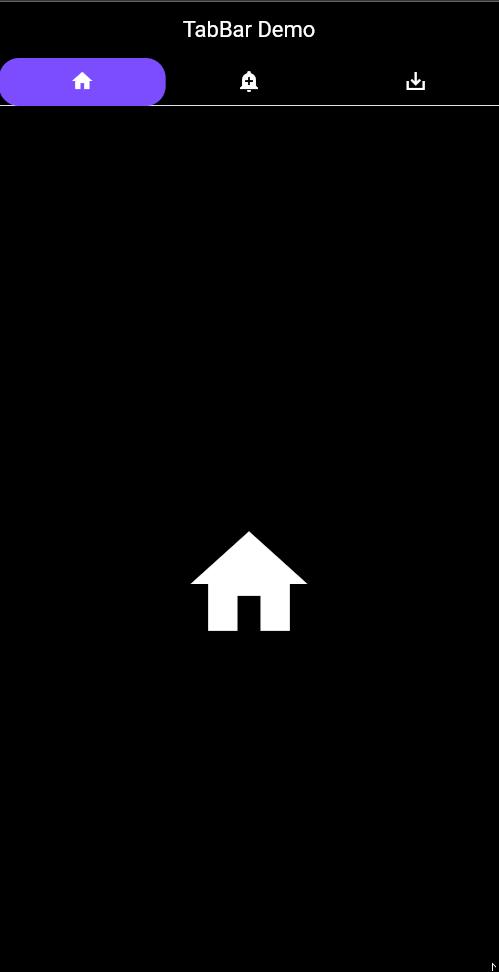
How to make scrollable tabs with TabBar?
Let’s imagine we are working on an app which has a lot of categories. To achieve this we have two types of scrolling i.e Horizontal and Vertical Scrolling.
Horizontally Scrollable Tabs
To achieve horizontal scrolling, the TabBar widget has a property to configure horizontally scrollable tabs. Use isScrollable to true that’s you are all set.
TabBar(
isScrollable: true,
tabs: []
)
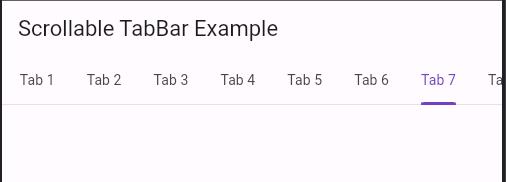
Conclusion
In this tutorial, we learned about the basics of the TabBar class in Flutter. We learned what is TabBar, and how it works. I hope this blog will be helpful for you to clarify the concept of TabBar in Flutter.